Back
Product News
Jul 16, 2023
How to send email attachments using nodemailer
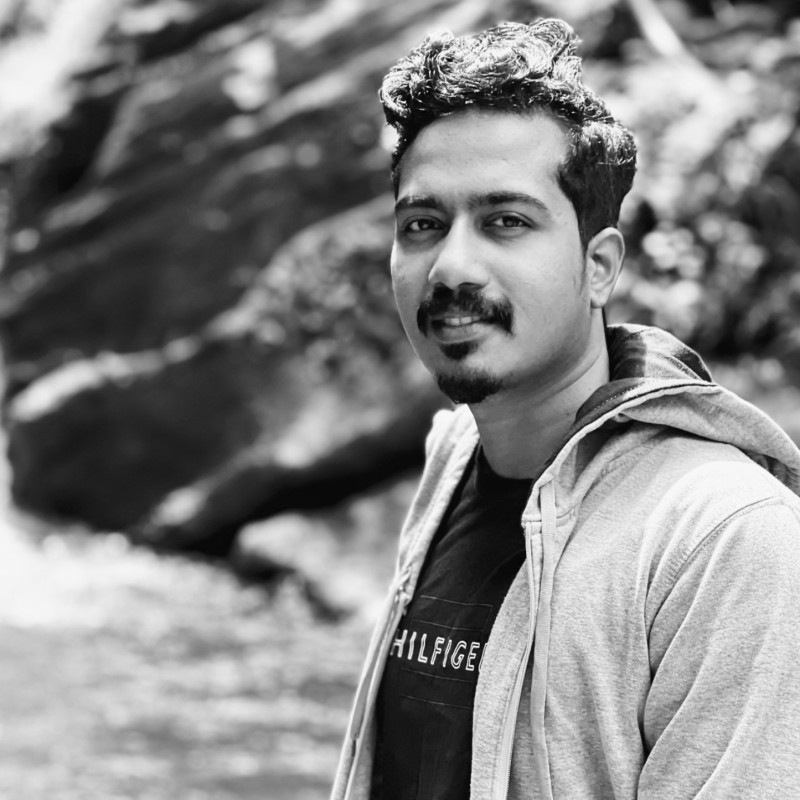
Anand Sukumaran
Nodemailer is a popular library used to send emails in Node.js. Features of nodemailer are
It has zero dependencies
Secure email delivery with SSL/TLS support
Supports email transport via popular providers like Amazon SES, Sendgrid etc.
Easy to add attachments unlike other libraries. In this tutorial we'll learn how to send email attachments using nodemailer. 1. First, add
nodemailer
to our project.
2. Now let's create a transport Like I mentioned above, nodemailer supports other thirdparty providers to be used as transport like Amazon SES, Sendgrid, Mailgun, SMTP etc. In this example, we'll use SMTP transport. I'll be using Typescript
for this example.
3. Construct the email body We can create the email body as an object with from
, to
, subject
and html
.
4. Let's add our attachment Nodemailer supports different types of attachments like base64
, file path, stream etc. To get the complete list of attachment supported by nodemailer, refer their documentation. In this example, we'll be using file path. So, let's modify our mailBody
.
5. Send the email To send the email, use the transport.sendMail()
function.
Final code to send email attachments using Nodemailer.
Simple! Hope this tutorial has helped you to understand how to send attachments using nodemailer in your email notifications.