Back
Tutorials
Jul 16, 2023
How to send emails in Nodejs using nodemailer [Guide Updated - 2024].
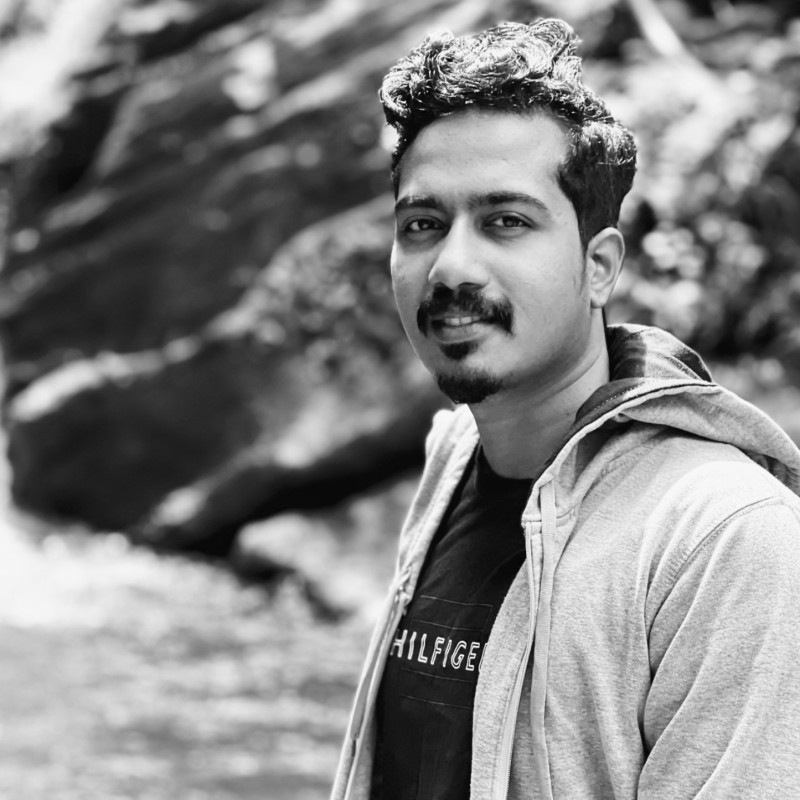
Anand Sukumaran
Sending emails from node.js app or an api can be easily done using a popular library called nodemailer. In this tutorial, we will learn how you can send emails from nodejs app using nodemailer and a popular transactional email provider such as Mailgun or sendgrid.
Options for sending email in Node.js using nodemailer.
As I mentioned above, nodemailer provides two options to deliver emails from node.js. One is using plain SMTP method, and the other one is using any email api service such as Amazon SES, mailgun, sengrid etc.
What is SMTP
SMTP is a universal protocol for delivering emails. Even if you use a third party email service, they will give you the SMTP credentials such as SMTP Host, Port, Email, Password etc that can be used with an SMTP client. While SMTP is easier to setup, it has some drawbacks over using an API to deliver emails. Email API providers add additional layers of security and performance optimization to enhance email delivery. It is also less prone to DDOS attacks and hacking. In this article, we will learn both methods to send emails in node.js using SMTP and API.
What is nodemailer transport
Nodemailer has this useful feature called transports, which is the "provider" that makes us able to send mail from node.js app. SMTP is a transport, Mailgun, or Sendgrid or similar services are another transport options.
Installing Nodemailer
Install Nodemailer using the following command:
OR
Once completed, include it into your web application
Sending email in Node.js using SMTP transport
One of the most common methods to send emails from a node.js app is to use the SMTP transport available in nodemailer. Now let's create the SMTP transport option in nodemailer. Use the SMTP credentials from your email provider. Almost all providers will generate an SMTP credential for you which could be accessed from their dashboard.