Back
Tutorials
Apr 10, 2024
How to create a service worker in a react application

Anand Sukumaran
Service worker is a script that works on the background of Javascript apps on a different thread. It essentially acts as a proxy between the web browsers and web servers. Service workers are used for multiple uses like enabling background sync, offline access, push notifications etc.
In this article, we'll learn how to setup and use a service worker in a React application, particularly for the purpose of enabling web push notifications. If you don't know what is web push notification, you can read this guide first.
How service worker work in a React application
It doesn't matter if your app is written in React or Angular or Vue. The working of service workers are the same in any Javascript application. Service worker is a Javascript file that can be accessed publicly (for eg: https://yourdomain.com/service-worker.js).
Service workers needs to be registered first. And the registration happens within a scope of your website. For example, if a service worker is registered under the scope https://yourdomain.com/sub-dir/
, the sub-dir
is the scope. One one service worker can be registered under a single scope.
Lifecycle of a service worker
The service worker lifecycle mainly consists of three stages. They are -
Registration
Installation
Activation
Registration phase involves registering the service-worker javascript file with your React application. Before that, we usually check if the browser supports service workers. Then call the navigator.serviceWorker .register()
function to register our Javascript file as the service-worker.
After the service worker is successfully registered, it is has not started to function yet. The Service worker script is downloaded and after that, the browser will try to install the service worker. This step is done immediately after the registration is completed.
Once installation is successful, the next step is to activate the service-worker. But unlike the installation step, the service worker is not immediately activated after installation.
The service worker will only be activated in any of these cases:
If there are no service workers that are currently active
If the
self.skipWaiting()
is called in theinstall
event handlerIf the page is refreshed by the user
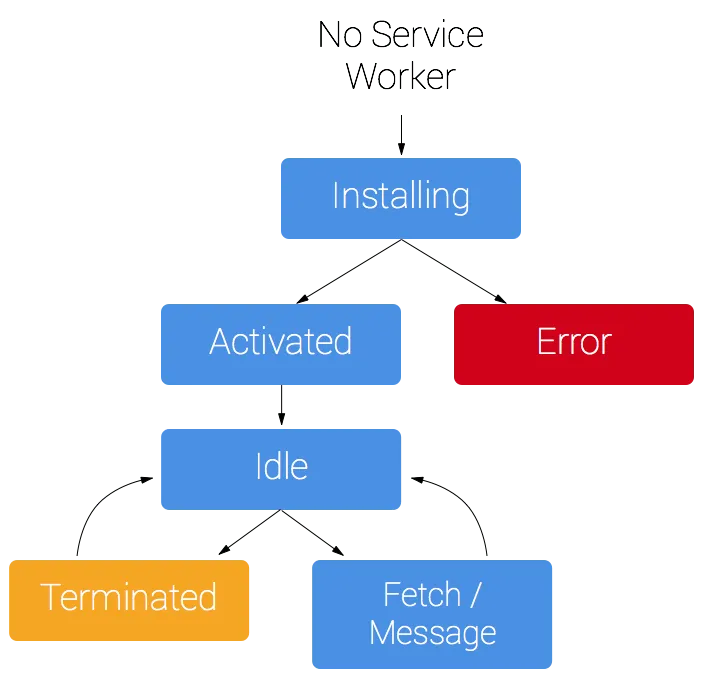
Creating the service worker file
Service worker file must live inside the public
directory of your React app. That means, it should be publicly accessible by anyone on your domain.
The filename can be anything like service-worker.js
or sw.js
.
Then make sure the script content is ready. For example, if you're planning to integrate web push notifications in your React app using Engagespot, this is the typical content you'd want to have in your service worker file.
Registering the service worker in React application
Depending on the build tool you're using (for eg: Vite), you may have different built-in configuration files to enable the service worker registration for your React app. In this article, we'll learn a tool agnostic way to setup service workers.
To register a service worker, you should have the following code somewhere on the <head>
section of your index.html
file of your React app.
Some key things to understand here.
Service workers will work only on HTTPS (except for localhost)
Syntax errors in service worker file will result in registration failure
Checking the status of service worker
Once you've added the code to register a service worker, visit your React app URL. This starts the service worker registration process.
If you want to check the status of service worker, open the developer tools
menu of your browser, and navigate to Application
tab. You can see the status there.
If you have made some changes to the service-worker.js
script, then you can perform an update by clicking on the Update
button.

Learn more about service workers
If you'd like to learn more about service workers and the use-cases, this service worker cookbook would be really helpful