Back
Tutorials
Jul 23, 2023
How to send emails via Amazon SES in Laravel along with in-app and push using a single SDK.
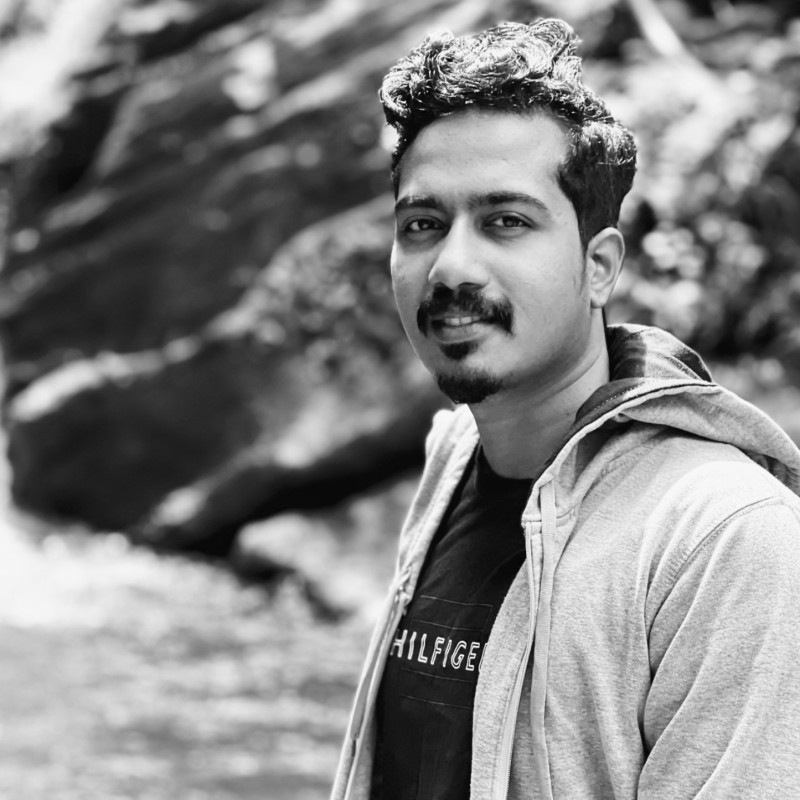
Anand Sukumaran
This article will move through a simple tutorial on how you can integrate email, in-app, and push notification features in your PHP Laravel application. To send we'll email notifications, we will use Amazon Simple Email Service (SES). To make this easier, and avoid integrating different APIs for each notification channel, we will use Engagespot multi-channel notification API.
Setting up Amazon SES
Amazon SES is a cloud-based transactional email service provider which allows users to send bulk quantity emails in a single go. Amazon SES, on its own, also accepts integration with applications or web-apps that allow for a smoother and in-app experience. The following details explain how you can connect your AWS SES service to your Engagespot to be further used in a laravel code.
Enabling SES through Engagespot
All you have to do is to simply log-in to your Engagespot account → Head over to Channels → Email → And now simply enable the listed AES SES Provider option.
Setting up Api Key and Secret using AWS IAM service
This will be the initialization of the connection between Engagespot and AWS SES. Head to your AWS SES dashboard and navigate to the IAM Service. Once done, navigate to Access management → Add Users and add a user. Now set the credential type to Access key - Programmatic Access. Navigate to the third section of Set permissions → Select the third option of Attach existing policies directly and click Create policy. Once the policy tab opens, select the JSON section and work the following code into the tab.
This will configure a policy for your SES service which depicts all the constraints and functions you will be using. Once done, locate the Tags button, hit it then locate the Review button and select it, now give a name to your policy and press on Create policy button. Now, navigate back to the Set permissions section, select the policy you just created and click on the Tags → Review button and finally click on Create user button. You will now be presented with an AWS Access Key ID
and Secret Key
, save both the values and proceed with the tutorial. Once your key ID and key is noted down, head over to your Engagespot Dashboard → Channels → Email → AWS SES. A pop-up will appear, enter the Key ID and the Secret Key in the relevant fields, enter your state/region in the Region field and enter the Verified Identity Email from your Engagespot account in the Email field.
What are channels in Engagespot?
Channels are the vessels through which the notification will be relayed to the relevant entity/visitor on your application, it can either be an Email, Web-Push, In-app or SMS channel. Enagespot takes up a multi-channel approach as multi-level vessels of communication are said to increase productivity and achieve consumer loyalty. This article also sheds light on how you can utilise these channels through Enagespot directly into your application or web-app.
Integrating AWS SES with Laravel
In order to integrate engagespot’s API with your application, you need to first set-up the working environment necessary for the changes in your application. Let's see how to do this
Installing the package
Installing the Engagespot package will allow the application to read and write through the API. You can use the jobin/engagespot package to send emails via aws ses. Use the following command in your app’s directory using the composer handler. composer require jobin/engagespot
This will install all the required prerequisites of the API.
Configuring service providers
Since we are using Engagespot’s service, we need to configure it appropriately in-order for the API to work through Engagespot in our application. Use the following command in the config/app.php file’s provider array.
This will initialise Engagespot as the primary service provider for the utility. In later versions of Laravel (5.5+), this is performed automatically.
Publishing the config file to enagespot
The provider is initialised but still won’t connect to Engagespot unless we publish your built config file, use the following command in the php CLI
Once published, the setup is almost done, you can now try sending requests and testing the system in itself.
Starting up and testing
Once your setup has successfully passed through, you can now open up your config/engagespot.php
file which has been newly created after publication. Add the API Key ID and Secret Key using the following syntax in the config/engagespot.php file.
Now, to send the notifications via Amazon SES Email, In-App and Push from your laravel code, just use the send()
function in Engagespot
class. You can try sending a notification and test the system out using the following block of code.